# 01 Random
# Intro to Random
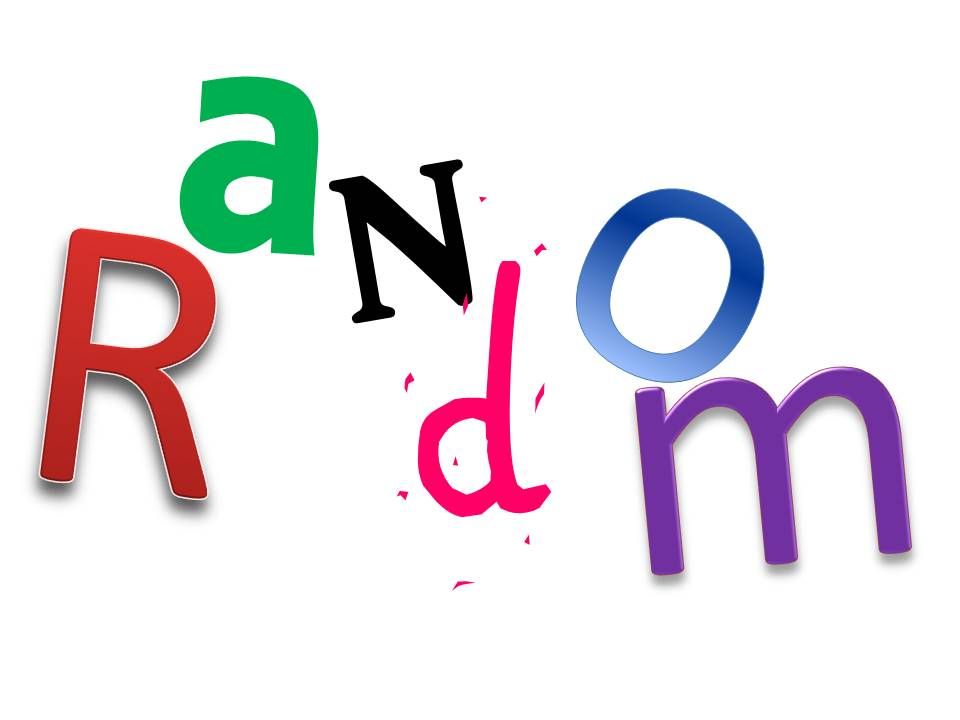
# Goal:
Learn how to use a Random object to generate random integers within specified number ranges.
# Steps:
- Generate a random integer without limits.
- Generate a random positive integer with an upper limit.
- Generate a random integer with an upper and lower (positive) limit.
- Generate a random integer with an upper and lower (negative) limit
# Rock Paper Scissors
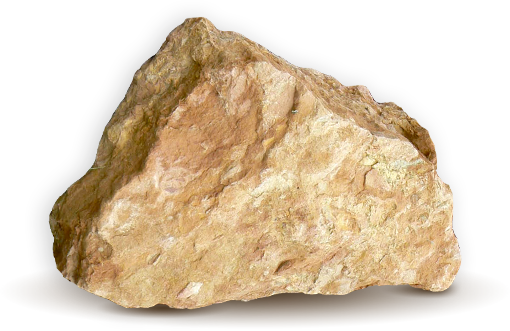
# Goal:
Use a Random object to generate random integers so your code can play Rock Paper Scissors with you.
# Steps:
- Create a Random object.
- Generate a random number to represent rock, paper, or scissors in a game.
- Play Rock Paper Scissors against the computer to test your code.
# Dice Roll
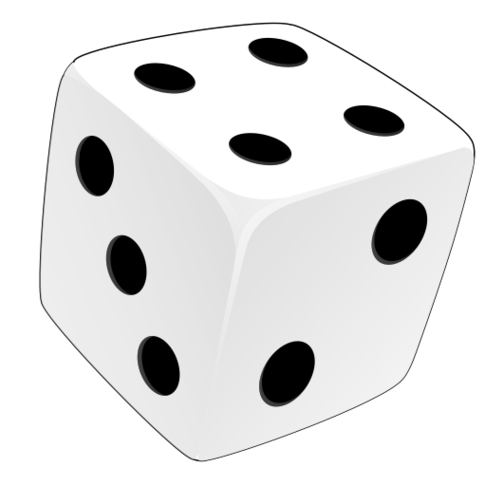
# Goal:
Use a Random object to generate random integers so your code can roll different numbers on a dice.
# Steps:
- Create a Random object.
- Generate a random number between 1 and 6.
- Change the code to display the dice image to match the number each time the "CLICK HERE TO ROLL" button is pressed.
- Test your code to make sure all 6 sides are displayed.
# Validation
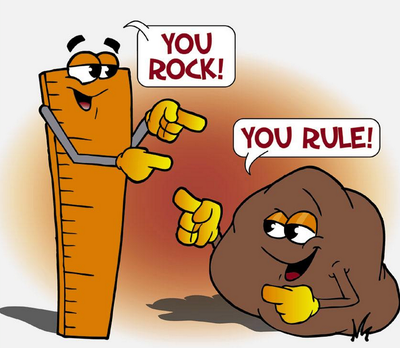
# Goal:
Use a Random object to generate random integers so your code can generate random compliments.
# Steps:
- Create a Random object.
- Generate a random number between 0 and 4.
- Map each value (0-4) to a unique compliment.
- Print a random compliment 10 times (using a loop)
- Test your code and receive some compliments.
# Magic 8 Ball
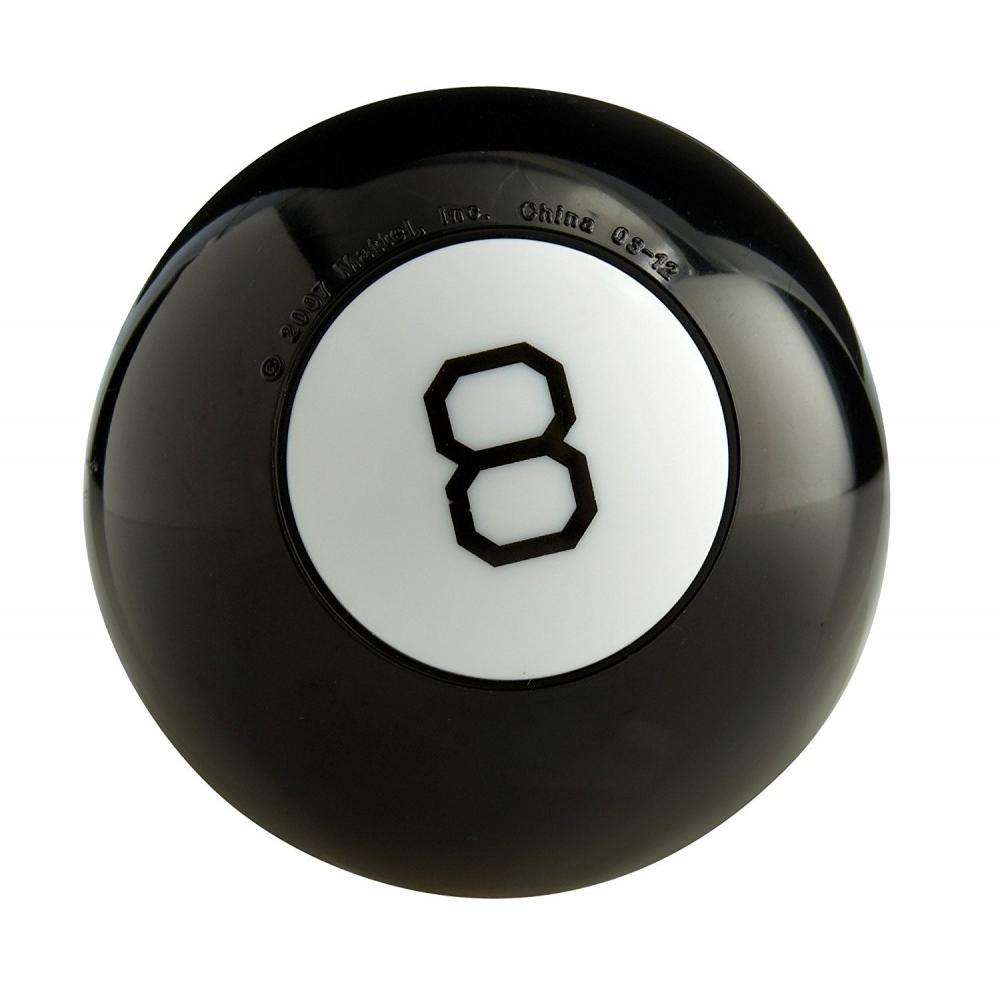
# Goal:
Use a Random object to generate random integers so your code can generate random messages from the Magic 8 Ball.
# Steps:
- Create a Random object.
- Generate a random number between 0 and 3.
- Map each value (0-3) to a unique message.
- Test your code and receive some magic answers from the 8 ball.
# Lottery Numbers
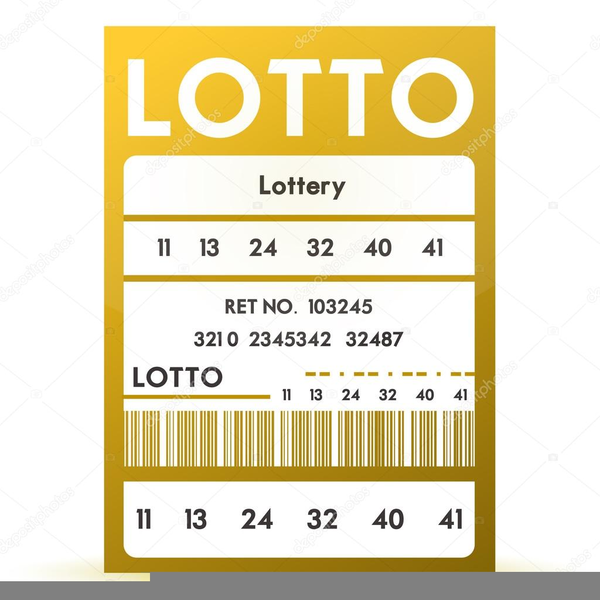
# Goal:
Use random numbers to make a lottery ticket!
# Steps:
- Add a new class for the Lottery Numbers recipe.
- Get 6 random numbers to put on your lottery ticket
- Display the selected numbers to the user in a pop-up (see below)
(All 6 random numbers must appear on the same pop-up [i.e. the user should not have to click "ok" 6 times]) 4. Bonus: set the title of the pop-up to show it is a lottery ticket.