# 01 Else If
# Robot Color Chooser
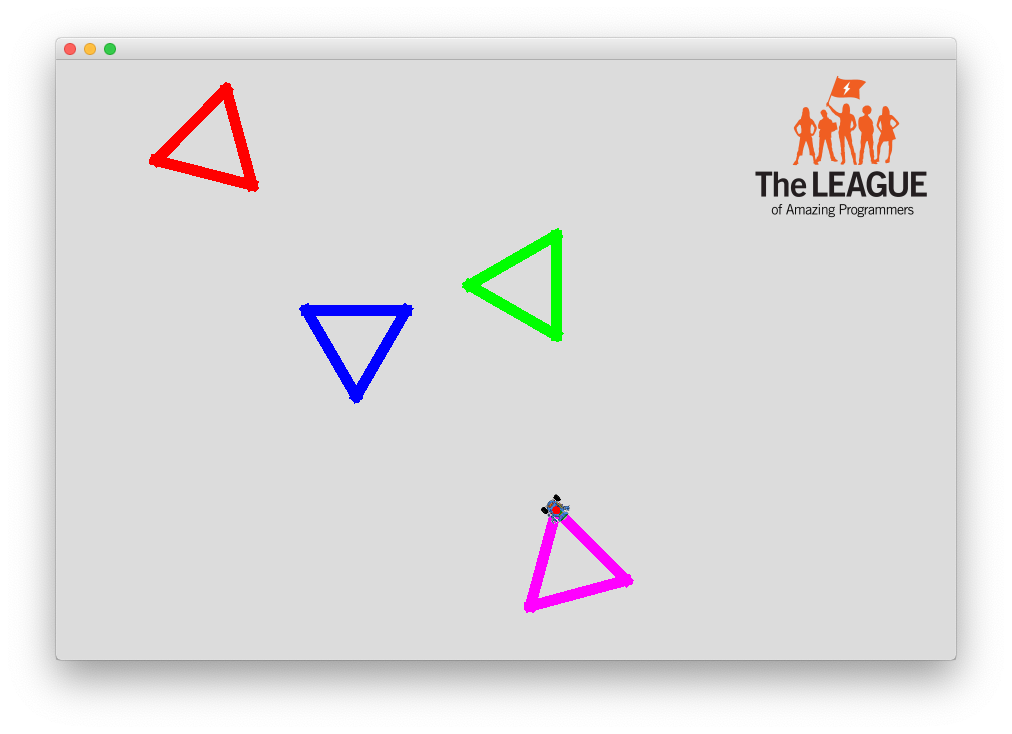
# Goal:
Use if and else if statements to set a Robot's pen to a chosen color before drawing a shape.
# Steps:
- Ask the user to choose a color.
- Set the Robot's pen to the chosen color.
- Have the Robot draw a shape.
- Repeat the above in a loop to test all the supported colors.
- Don't forget to use an else statement in case the user enters an unrecognized color.
# Crazy Cat Lady
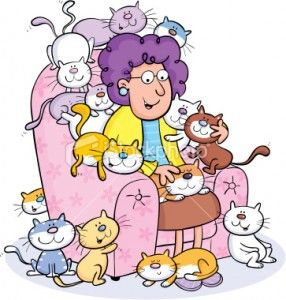
# Goal:
Use if and else if statements to provide different responses to a user based on how they answer the question, "How many cats do you have?".
# Steps:
- Ask the user how many cats they have.
- Convert their answer into a number (int).
- If they have 3 or more cats, they might be a crazy cat lady.
- If they have less than 3 cats AND more than 0 cats, they are a cat lover.
- If they have 0 cats, maybe they have a different pet.
# High Low Game
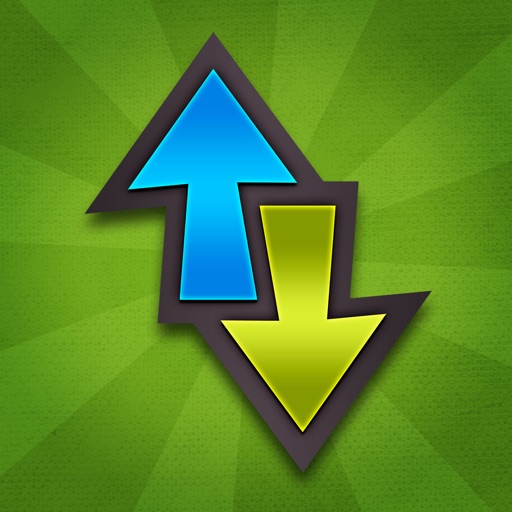
# Goal:
Use if and else if statements in a loop until the user correctly guesses the secret number in a high low game.
# Steps:
- Get a random number between 1 and 100. Hint: use a Random object
- Ask the user to guess what the number is.
- If their guess is correct, tell them they won and end the game.
- If their guess is too high, tell them it is too high.
- If their guess is too low, tell them it is too low.
- Use a for loop to repeat the above code 10 times. If they don't find the number after 10 tries, tell them they lost the game and tell them the number.
# Are You Happy?
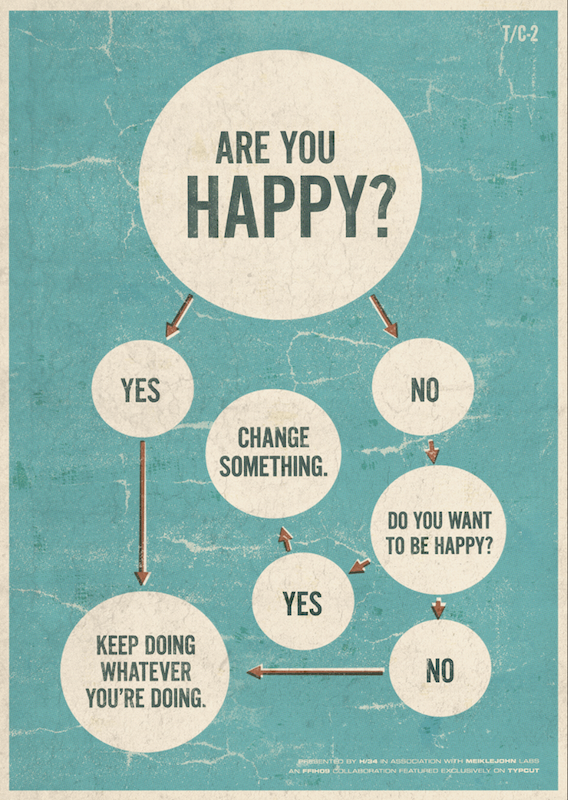
# Goal:
Create a program that mimics the chart above using if and else if statements.
# Steps:
- Use JOptionPanes and if / else if /else statements to recreate this chart.
# Choose Your Own Adventure

# Goal:
Tell the user a story, but give them options so they can decide the path of the plot.
# Steps:
- Use JOptionPanes, if statements, and your imagination to make an interesting story.