# 01 Algorithms
# Prime or Not
# Goal:
Ask the user for a number, then tell them if the number is prime! A prime number is a number that is only divisible by 1 and itself.
# Steps:
- Ask the user for a number (JOptionPane).
- Use a for loop, if statement, and modulo to find if the number is prime.
- If the number is divisible by any number other than 1 or itself, the number is not prime.
# Fibonacci
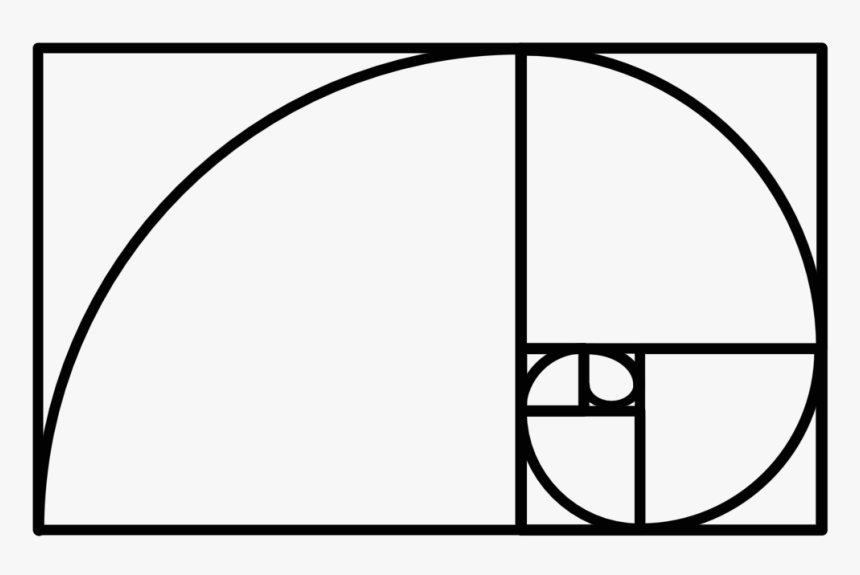
# Goal:
Print out the first 12 numbers of the fibonacci sequence.
# Steps:
- There is more than one way to code a solution to this. The following steps give you some guidelines for one of them.
- Declare and initialize three int variables: number1, number2, and sum.
- Initialize number1 and number2 to the first two numbers of the fibonacci sequence (0 and 1) and print both numbers.
- Use a for loop that calculates the sum of the two numbers and prints it. The for loop should repeat 10 times.
- Now try to figure out how to change the variables before the for loop repeats so the sequence of numbers is correct.
# Goofy Names
GoOfY NaMeS
# Goal:
Write an algorithm to change a String into a "goofy" version.
# Steps:
- Ask the user to enter their name.
- Use a loop to alternately modify each character of the name into uppercase and lowercase letters until a new "goofy" representation of their name has been constructed.
For example, if they enter their name as Alexander Hamilton
their goofy name will be AlExAnDeR HaMiLtOn 3. Show the user the goofy version of their name in a pop-up.